Mostly, there are multiple ways to write a script to perform a single task. It varies from Developers to Developers, who normally try to follow the best practice. But sometimes some standards discard from time to time (version to version). In this article, I will go through some standard formats that we used in the prior version of SQL server but does not work in SQL Server 2012. It may break your script.
Let me create a sample in SQL Server 2005/ 2008 to explain it.
USE [test]
GO
CREATE TABLE [dbo].[t_Student](
[Student ID] [int] IDENTITY(1,1) NOT NULL,
[Student Name] [nvarchar](50) NULL
)
After creating the sample table lets alter this table. But there are three different formats that you can use to alter the table in earlier version of SQL server (2005/2008)
Method 1 :
In method 1, the format to alter the table will be .Database Name.Schema.Table Name
Alter table .[test].dbo.[t_Student] Add [Contact Details] varchar(50) NULL
--OUTPUT
Command(s) completed successfully
Method 2 :
In method 2, the format to alter the table will be ..Schema.Table Name
use test
GO
Alter table ..dbo.[t_Student] Add [Date of Birth] Datetime NULL
--OUTPUT
Command(s) completed successfully
Method 3 :
In method 3, the format to alter the table will be Schema.Table Name
use test
GO
Alter table dbo.[t_Student] Add [Date of Admission] Datetime NULL
--OUTPUT
Command(s) completed successfully
All of the above methods executed successfully in SQL Server 2005/ 2008.
Lets browse the table.
Select * from t_Student
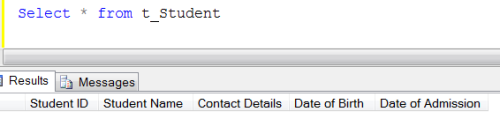
Now, lets do the same exercise in SQL Server 2012.
Method 1 :
In method 1, the format to alter the table will be .Database Name.Schema.Table Name
Alter table .[test].dbo.[t_Student] Add [Contact Details] varchar(50) NULL
--OUTPUT
Msg 117, Level 15, State 1, Line 1
The object name ‘.test.dbo.t_Student’ contains more than the maximum number of prefixes. The maximum is 2.
Method 2 :
In method 2, the format to alter the table will be ..Schema.Table Name
use test
GO
Alter table ..dbo.[t_Student] Add [Date of Birth] Datetime NULL
--OUTPUT
Msg 117, Level 15, State 1, Line 1
The object name ‘..dbo.t_Student’ contains more than the maximum number of prefixes. The maximum is 2.
Method 3 :
In method 3, the format to alter the table will be Schema.Table Name
use test
GO
Alter table dbo.[t_Student] Add [Date of Admission] Datetime NULL
--OUTPUT
Command(s) completed successfully
Conclusion :
If you are planning to upgrade your SQL Server earlier version to SQL Server 2012 or you are using SQL Server 2012, remember, it will not allow you to use methods 1 & 2. In case your are using above methods 1 & 2 in Dynamic SQL or Static SQL, it may break your script.
Reference : MSDN
Read Full Post »